Often you want to save user input in a database.
As you've probably already figured out, this can be done by creating a form as described in lesson 11 - where the values from the form fields can be inserted in the SQL statement. Suppose you have a simple form like this:
<form action="insert.php" method="post"> <input type="text" name="FirstName" /> <input type="submit" value="Save" /> </form>
The form submits to the file insert.php where you, as shown in lesson 11, can get the user's input by requesting the form content. In this particular example, an SQL statement could look like this:
strSQL = "INSERT INTO people(FirstName) values('" . $_POST["FirstName"] . "')"
In the same way, it is possible to retrieve data from cookies, sessions, query strings, etc.
Most common beginner mistakes
In the beginning, you will probably get a lot of error messages when you try to update your databases. There is no room for the slightest inaccuracy when you work databases. A misplaced comma can mean the database is not being updated, and you get an error message instead. Below, we describe the most common beginner mistakes.
Wrong data types
It is important that there is consistency between the type of data and column. Each column can be set to a data type. The screenshot below shows the data types for the table "people" in our example.
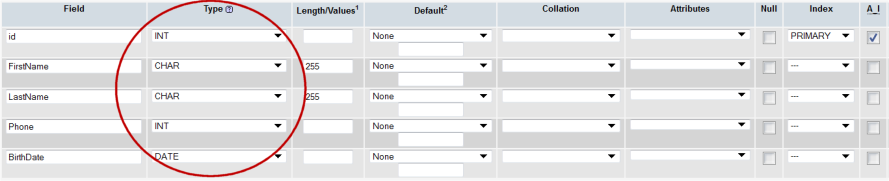
An error occurs if you, for example, attempt to insert text or numbers in a date field. Therefore, try to set the data types as precisely as possible.
Below is the most common data types listed:
Setting | Data Type | Size |
---|---|---|
CHAR | Text or combinations of text and numbers. Can also be used for numbers that are not used in calculations (e.g., phone numbers). | Up to 255 characters - or the length defined in the "Length" |
TEXT | Longer pieces of text, or combinations of text and numbers. | Up to 65,535 characters. |
INT | Numerical data for mathematical calculations. | 4 bytes. |
DATE | Dates in the format YYYY-MM-DD | 3 bytes. |
TIME | Time in the format hh:mm:ss | 3 bytes. |
DATETIME | Date and time in the format YYYY-MM-DD hh:mm:ss | 8 bytes. |
SQL statements with quotes or backslash
If you try to insert text that contains the characters single quote ('), double quote (") or backslash (\), the record may not be inserted into the database. The solution is to add backslashes before characters that need to be quoted in database queries.
This can be done with the function
addslashes this way:

<?php $strText = "Is your name O'Reilly?"; $strText = addslashes($strText); ?>
All single quotes ('), double quotes (") and backslashs (\) will then get an extra backslash before the character. This would only be to get the data into the database, the extra \ will not be inserted. Please note that PHP runs
addslashes on all $_GET, $_POST, and $_COOKIE data by default. Therefore do not use
addslashes on strings that have already been escaped.


In the next lesson you will learn to retrieve data from your database. But first, try to insert some more people in your database (as shown in the example above with Gus Goose).
No comments:
Post a Comment